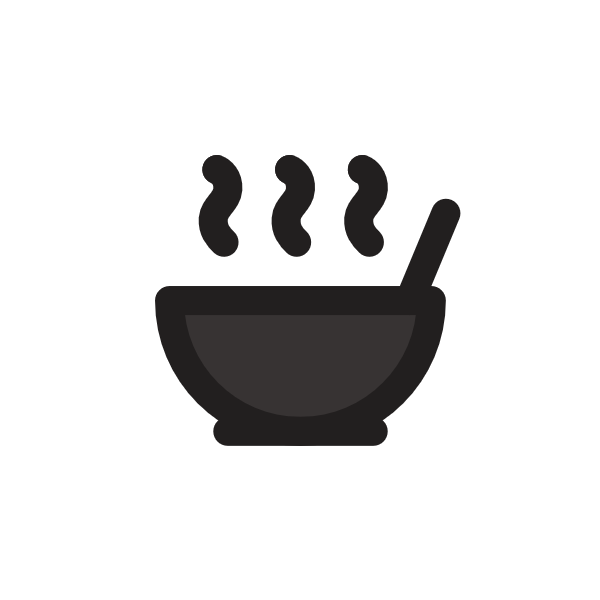
Cocojunk
🚀 Dive deep with CocoJunk – your destination for detailed, well-researched articles across science, technology, culture, and more. Explore knowledge that matters, explained in plain English.
Heuristic (computer science)
Read the original article here.
Heuristics: The Shortcuts, Guesses, and Black Magic of Real-World Code
In the world of textbook algorithms, you're often taught methods that guarantee finding the perfect solution. They are elegant, provably correct, and beautiful in their mathematical purity. But step outside the classroom, into the chaotic, resource-constrained reality of building systems that actually work within deadlines and on finite hardware, and you quickly learn a secret: perfect is often the enemy of good enough.
This is where heuristics come in. They are the pragmatic shortcuts, the educated guesses, the "forbidden" techniques that allow you to tackle problems too complex, too large, or too time-sensitive for traditional, exact methods. They trade guaranteed optimality for speed and practicality. While they might not always find the best answer, they find an answer – often a very good one – when the "proper" methods would simply fail or take eons. Mastering heuristics is a crucial part of the underground programmer's toolkit.
What is a Heuristic? (The Pragmatic Definition)
Forget absolute certainty for a moment. A heuristic is about making progress, quickly, even when you don't have all the information or the time for a perfect analysis.
Heuristic: In computer science and mathematical optimization, a technique designed to solve a problem more quickly when classic methods are too slow, fail entirely, or require impractical resources. This speed is achieved by trading off guarantees of optimality, completeness, accuracy, or precision. Think of it as a clever shortcut or rule of thumb that usually works well in practice.
And often, this shortcut is guided by a specific function:
Heuristic Function (or simply, Heuristic): A function used within a search or optimization algorithm to estimate how close a given state or option is to the goal or optimal solution. It helps the algorithm decide which path or option is most promising to explore next, without needing to compute the exact cost or outcome.
The core idea is: get a good result now instead of waiting forever for the perfect result.
Motivation: Why Go Underground with Heuristics?
Why deviate from the path of guaranteed solutions? The real world is messy.
- Speed is Paramount: Many problems, especially in real-time systems, simulations, or large-scale data processing, simply cannot afford the time it takes for an algorithm to explore every possibility or guarantee the absolute best outcome. A quick, decent solution today is better than a perfect solution next year.
- Handling Intractable Problems: Theoretical computer science reveals that many important problems belong to a class known as NP-hard. For these problems (like the Traveling Salesman Problem, complex scheduling, many optimization tasks), the time required to find the guaranteed optimal solution grows exponentially with the size of the problem. Even with the most powerful computers, you hit a wall very quickly. Heuristics are often the only viable way to get any solution for practical instances of these problems.
- Bootstrapping Other Algorithms: Sometimes, a heuristic doesn't solve the problem entirely but provides a good starting point or "seed value" for more rigorous optimization algorithms, helping them converge faster or find better solutions than they might starting from scratch.
- Navigating Unknown Territory (AI & Simulation): In artificial intelligence, game playing, and complex simulations, you often encounter situations where there's no predefined algorithm for the optimal action. Heuristics provide a way for the system to evaluate potential moves or states based on available information and make a reasonable choice, mimicking intelligent decision-making. They allow systems to "find" solutions in vast, complex "search spaces."
In essence, heuristics are born out of necessity when academic purity clashes with practical constraints. They are the tools you use when the rulebook doesn't provide a fast enough answer, or any answer at all.
Why "Forbidden"? The Perilous Power
Heuristics aren't typically the first thing you learn in a fundamental algorithms class because they lack those beautiful guarantees. This is their "forbidden" aspect – they operate outside the realm of provable perfection.
- Lack of Optimality Guarantee: A standard algorithm might guarantee the shortest path; a heuristic might find a short path, but not necessarily the shortest.
- Lack of Completeness Guarantee: Some heuristics might lead you down a path where no solution is found, even if one exists.
- Empirical vs. Theoretical: Many powerful heuristics are developed through trial and error, experience, and observation ("rules of thumb") rather than derived from rigorous mathematical theory. They work because they have been seen to work, not necessarily because they must work in all cases.
Understanding and accepting these limitations while leveraging the power is key to using heuristics effectively in the "underground."
Examples: Heuristics in Action
Let's look at some concrete examples of how heuristics are applied, from classic problems to modern software.
1. Solving a Simpler Problem (Relaxation)
One common heuristic technique is to simplify the problem in a way that makes it solvable quickly, and then use the solution to the simplified problem as a guide or an approximate solution for the original, complex one.
Concept: Identify the core difficulty in the problem and remove or relax constraints until it becomes easy. The solution to the relaxed problem provides a heuristic estimate for the original.
Example: Pathfinding on a Grid
Imagine you're trying to find the shortest path between two points on a complex map with obstacles. A "simple" heuristic might be the straight-line distance (Euclidean distance) or the Manhattan distance (horizontal + vertical distance) between your current location and the goal.
- Original Problem: Find the shortest path navigating around walls, varied terrain costs, etc. (Complex)
- Simplified Problem (Heuristic): Ignore all obstacles and terrain. Calculate the direct "as-the-crow-flies" distance. (Simple)
This simple distance calculation (the heuristic function h(current_node, goal_node)
) doesn't account for walls or path difficulties, but it gives you a quick estimate of how far you might still need to travel. Algorithms like A* use this heuristic to prioritize exploring nodes that seem closer to the goal according to this simple estimate.
2. The Traveling Salesman Problem (Greedy Approach)
The Traveling Salesman Problem (TSP) is a classic NP-hard problem:
Travelling Salesman Problem (TSP): Given a list of cities and the distances between each pair, find the shortest possible route that visits each city exactly once and returns to the origin city.
Finding the absolute shortest path requires checking a huge number of possibilities (specifically, (n-1)! / 2 unique tours for n cities - a massive number even for relatively small n). This is computationally infeasible for even moderately sized problems (e.g., 50 cities).
Heuristic Solution (Greedy Algorithm): A common heuristic for TSP is the "Nearest Neighbor" or greedy approach.
- Start at a random city.
- From the current city, always move to the unvisited city that is closest.
- Repeat until all cities are visited.
- Finally, return to the starting city.
Why it's a Heuristic: This algorithm makes decisions based purely on immediate gain (the shortest edge right now) without considering the long-term consequences (like potentially isolating a distant city that's hard to reach later).
Trade-off: It's extremely fast. For n
cities, it's roughly an O(n²) operation. However, the resulting tour is often not the globally shortest one. You might pick a close city early that forces a much longer detour later.
Real-World Use Case: Planning delivery routes, optimizing tool paths for manufacturing machines, scheduling tasks. While not perfect, the greedy solution is fast enough for many practical applications and provides a tour that's reasonably good, far better than a random route.
3. Guiding Search (Alpha-Beta Pruning, A*)
Heuristics are fundamental to making search algorithms efficient, especially in AI and combinatorial problems.
Concept: Instead of exhaustively searching every possible path or state (like Breadth-First Search or Depth-First Search might initially), heuristics help decide which branches of the search space are most promising to explore first or which branches can be safely ignored.
Alpha-Beta Pruning (Game AI): Used in game-playing algorithms (like for Chess or Go). It's a heuristic technique that cuts off branches in the game tree that evaluation determines are not going to lead to a better result than one already found. You don't need to analyze a losing line of play in detail if you've already found a winning one. The heuristic here is the evaluation function that estimates the value of a game state for one player.
A Search (Pathfinding):* A* is a best-first search algorithm that finds the shortest path between a start and goal node in a graph. It uses a heuristic function (
h(n)
) along with the actual cost incurred so far (g(n)
) to prioritize exploration. It evaluates nodes based onf(n) = g(n) + h(n)
, wheref(n)
is the estimated total cost of the path through noden
.The heuristic
h(n)
provides the "guess" about the remaining cost to the goal. This guidance significantly speeds up the search compared to algorithms that only consider past cost (g(n)
).Admissible Heuristic: A heuristic
h(n)
is admissible if it never overestimates the cost to reach the goal from noden
. Formally,h(n) <= d*(n, goal)
, whered*(n, goal)
is the true shortest path cost fromn
to the goal. For algorithms like A* searching for the optimal path, using an admissible heuristic is crucial because it guarantees that the first time the algorithm reaches the goal node, it will be via the shortest path.Example: In grid pathfinding, Manhattan distance and Euclidean distance are admissible heuristics (assuming movement costs are not zero or negative). You can't possibly reach the goal in fewer steps than the straight-line or grid distance.
4. Newell and Simon: The Heuristic Search Hypothesis
Pioneers of AI, Allen Newell and Herbert A. Simon, formalized the idea that intelligent problem solving often relies on heuristic search.
Concept: They proposed that intelligent systems solve problems by generating potential solutions (symbol structures) and then evaluating them using heuristics to guide the process, modifying the structures based on how close they are to a desired state (the solution). This isn't random trial-and-error; it's informed trial-and-error.
Mechanism: Using a search tree representation of the problem space, instead of exploring every single possible state or sequence of actions, the system uses heuristics to select and prioritize branches that seem most likely to lead to the solution. It prunes away or ignores branches that the heuristic suggests are unpromising.
Implication: This hypothesis underscores that much of what we perceive as intelligent behavior – from playing chess to solving logical puzzles – can be modeled as a heuristic-guided search through a vast space of possibilities. It's a departure from the idea of pre-programmed perfect algorithms; instead, it's a dynamic process of exploration guided by experience or learned evaluation rules (heuristics).
5. Antivirus Software: Detecting the Unknown
This is a prime example of heuristics in action against evolving threats.
Traditional Antivirus: Relies heavily on signatures – exact patterns of known viruses. Fast and accurate for known threats, but useless against new or modified ones.
Heuristic Antivirus: Uses rules and patterns based on behavior or characteristics common to malicious software, rather than specific signatures.
Concept:
- Look for code patterns associated with self-modification (polymorphism).
- Monitor process behavior: Does a program try to write directly to system files? Does it attempt to encrypt large numbers of files? Does it try to open network ports unexpectedly?
Why it's a Heuristic: The rules are based on observed tendencies of malware. A program exhibiting these behaviors is likely malicious, but not guaranteed. A legitimate program could theoretically trigger some heuristic rules (a false positive).
Trade-off: Can detect new or mutated viruses that have no known signature (powerful "underground" capability!). However, it has a higher potential for false positives (identifying legitimate software as malware) compared to signature scanning.
Benefit: Allows detection of zero-day threats (attacks exploiting unknown vulnerabilities) and highly evasive malware that constantly changes its code (polymorphic/metamorphic viruses). This proactive capability is essential in a constantly shifting threat landscape.
Pitfalls: When Shortcuts Lead You Astray
The power of heuristics comes with significant risks. Because they sacrifice guarantees, they can sometimes lead to suboptimal solutions, no solution at all, or outright incorrect results. Understanding these pitfalls is crucial for the responsible "underground" developer.
Lack of Theoretical Foundation: Some heuristics are brilliant insights based on deep understanding or extensive experimentation. Others are merely educated guesses or "rules of thumb" that seem to work. Without a strong theoretical basis, you don't truly understand why it works or, more importantly, when it might fail.
Overfitting to Data: A heuristic developed based on a specific set of past data might perform poorly or fail entirely when applied to new data that doesn't follow the same patterns. This is a common problem when heuristics are derived purely empirically without considering the underlying problem structure.
Suboptimality: By definition, heuristics don't guarantee the best solution. While a "good enough" solution is often sufficient, there are times when missing the true optimum can have significant costs.
Incompleteness/Getting Stuck: A heuristic might guide a search algorithm down a path that leads to a dead end or a local optimum, missing the true goal or the global optimum. This is particularly a risk with "greedy" heuristics.
- Example (Non-Admissible Heuristic in Search): If a search heuristic overestimates the cost to the goal (i.e., it's not admissible), it might incorrectly prioritize exploring nodes that are further away, potentially missing the optimal shortest path altogether or getting stuck in cycles or undesirable parts of the search space.
Admissibility (Revisited): For a search heuristic
h(v_i, v_g)
estimating the distance to the goalv_g
from nodev_i
, it is admissible ifh(v_i, v_g) <= d*(v_i, v_g)
for all nodesv_i
, whered*(v_i, v_g)
is the true shortest distance. An inadmissible heuristic can make an algorithm like A* abandon the optimal path if it finds a suboptimal path that appears better based on the faulty, overestimating heuristic.Sensitivity: The performance of a heuristic can be highly sensitive to the specific parameters used or minor variations in the input data. What works perfectly for one instance of a problem might fail catastrophically for another.
Difficulty in Analysis: Because heuristics lack formal guarantees, analyzing their performance (how good the solution is, how fast it runs) is often done empirically or probabilistically, rather than through deterministic mathematical proofs.
Using heuristics requires a pragmatic mindset and careful testing. You must be aware of the trade-offs and validate that the heuristic performs acceptably for the range of inputs you expect to encounter.
Related "Underground" Techniques
Heuristics form the basis for many more advanced, pragmatic problem-solving techniques:
- Metaheuristics: Algorithms that provide a high-level framework for developing more specific heuristic algorithms. They often involve managing and combining multiple heuristics, using concepts like memory (keeping track of good solutions) and learning (adapting the heuristic over time). Examples include Genetic Algorithms, Simulated Annealing, Tabu Search, and Ant Colony Optimization. These are like meta-strategies for finding and refining heuristics.
- Matheuristics: Techniques that blend metaheuristics with exact mathematical programming (MP) techniques (like linear programming, integer programming). They leverage the speed of heuristics and the power of exact solvers together, often using one to improve the other.
- Reactive Search Optimization: Methods that use online machine learning principles to automatically tune the parameters of a heuristic during the search process itself, reacting to the characteristics of the specific problem instance being solved.
These related fields demonstrate the evolution of heuristics from simple rules of thumb to sophisticated, self-adapting systems for tackling the hardest computational problems.
Conclusion: Embracing the Shortcut
Heuristics are not a sign of computational weakness; they are a sign of computational savvy in the face of impossible odds. They are the essential tools for the underground programmer who needs to ship working solutions in the real world.
While they lack the clean mathematical proofs of their theoretical counterparts, heuristics empower you to:
- Solve problems that are otherwise intractable within practical constraints.
- Build intelligent systems that can navigate complex, uncertain environments.
- Deliver timely, if not absolutely perfect, results.
Mastering heuristics means understanding the problem deeply enough to devise effective shortcuts and, critically, understanding the pitfalls well enough to use them responsibly. They are a vital part of the "forbidden code" – the techniques necessary when the textbook isn't enough, and you just need to make things work.